The Facade pattern is a simple code structure laid over a more complex structure, in order to hide the complexity from the code which uses the facade.
The idea is that if you don't want other code accessing the complex bits of a class or process, you hide those bits by covering them with a Facade.
NOTE: This post is part of a series demonstrating software design patterns using C# and .NET. The patterns are taken from the book Design Patterns by the Gang of Four. Check out the other posts in this series!
The Rundown
- Type: Structural
- Useful? 5/5 (Extremely)
- Good For: Hiding complexity which cannot be refactored away.
- Example Code: On GitHub
The Participants
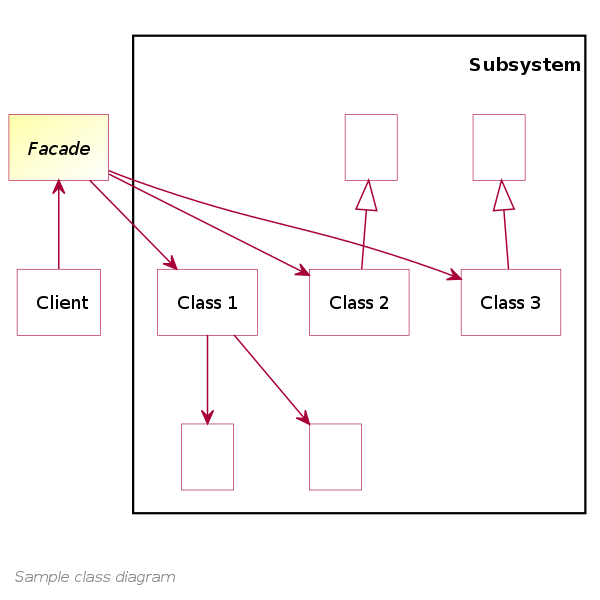
- The Subsystems are any classes or objects which implement functionality but can be "wrapped" or "covered" by the Facade to simplify an interface.
- The Facade is the layer of abstraction above the Subsystems, and knows which Subsystem to delegate appropriate work to.
A Delicious Example
To demonstrate how we use the Facade pattern, let's think about a restaurant.
In most kitchens, the work area is divided into sections. For this post, we'll assume that our kitchen is divided into three areas:
- Hot Prep, where hot dishes like meats and pasta are made
- Cold Prep, where cold dishes like salads and desserts are made, and
- The Bar, where drinks are prepared.
If you are a patron at this restaurant and you sit down at a booth, do you care what part of your meal is made at what section of the restaurant? Of course not. There is a natural layer of abstraction in place between you as the patron and the restaurant kitchen: the server.
The server knows where to place each order and where to pick those parts of the order up from. We'll model this relationship to demonstrate how the Facade pattern can simplify the structure of our code.
First, let's create a class Patron
for the restaurant patron:
/// <summary>
/// Patron of the restaurant (duh!)
/// </summary>
class Patron
{
private string _name;
public Patron(string name)
{
this._name = name;
}
public string Name
{
get { return _name; }
}
}
Let's also define the following:
- a base class
FoodItem
representing all food items sold at this restaurant, - an interface
IKitchenSection
representing all sections of this restaurant's kitchen, and - a class
Order
representing a patron's order:
/// <summary>
/// All items sold in the restaurant must inherit from this.
/// </summary>
class FoodItem { public int DishID; }
/// <summary>
/// Each section of the kitchen must implement this interface.
/// </summary>
interface IKitchenSection
{
FoodItem PrepDish(int DishID);
}
/// <summary>
/// Orders placed by Patrons.
/// </summary>
class Order
{
public FoodItem Appetizer { get; set; }
public FoodItem Entree { get; set; }
public FoodItem Drink { get; set; }
}
Now we can start to model the sections of the kitchen, AKA the Subsystem participants. Here's the classes for ColdPrep
, HotPrep
, and Bar
:
/// <summary>
/// A division of the kitchen.
/// </summary>
class ColdPrep : IKitchenSection
{
public FoodItem PrepDish(int dishID)
{
//Go prep the cold item
return new FoodItem()
{
DishID = dishID
};
}
}
/// <summary>
/// A division of the kitchen.
/// </summary>
class HotPrep : IKitchenSection
{
public FoodItem PrepDish(int dishID)
{
//Go prep the hot entree
return new FoodItem()
{
DishID = dishID
};
}
}
/// <summary>
/// A division of the kitchen.
/// </summary>
class Bar : IKitchenSection
{
public FoodItem PrepDish(int dishID)
{
//Go mix the drink
return new FoodItem()
{
DishID = dishID
};
}
}
Finally, we need the actual Facade participant, which is our Server
class:
/// <summary>
/// The actual "Facade" class, which hides the
/// complexity of the KitchenSection classes.
/// After all, there's no reason a patron
/// should order each part of their meal individually.
/// </summary>
class Server
{
private ColdPrep _coldPrep = new ColdPrep();
private Bar _bar = new Bar();
private HotPrep _hotPrep = new HotPrep();
public Order PlaceOrder(Patron patron,
int coldAppID,
int hotEntreeID,
int drinkID)
{
Console.WriteLine("{0} places order for cold app #"
+ coldAppID.ToString()
+ ", hot entree #" + hotEntreeID.ToString()
+ ", and drink #" + drinkID.ToString() + ".");
Order order = new Order();
order.Appetizer = _coldPrep.PrepDish(coldAppID);
order.Entree = _hotPrep.PrepDish(hotEntreeID);
order.Drink = _bar.PrepDish(drinkID);
return order;
}
}
Will all of these in place, we can use the Main()
method to show how a patron might place an order and how the server (the Facade) would direct the appropriate pieces of that order to the kitchen sections (the Subsystems):
static void Main(string[] args)
{
Server server = new Server();
Console.WriteLine("Hello! I'll be your server today. What is your name?");
var name = Console.ReadLine();
Patron patron = new Patron(name);
Console.WriteLine("Hello " + patron.Name
+ ". What appetizer would you like? (1-15):");
var appID = int.Parse(Console.ReadLine());
Console.WriteLine("That's a good one. What entree would you like? (1-20):");
var entreeID = int.Parse(Console.ReadLine());
Console.WriteLine("A great choice! Finally, what drink would you like? (1-60):");
var drinkID = int.Parse(Console.ReadLine());
Console.WriteLine("I'll get that order in right away.");
//Here's what the Facade simplifies
server.PlaceOrder(patron, appID, entreeID, drinkID);
Console.ReadKey();
}
If we run the sample app, an output might look like this:
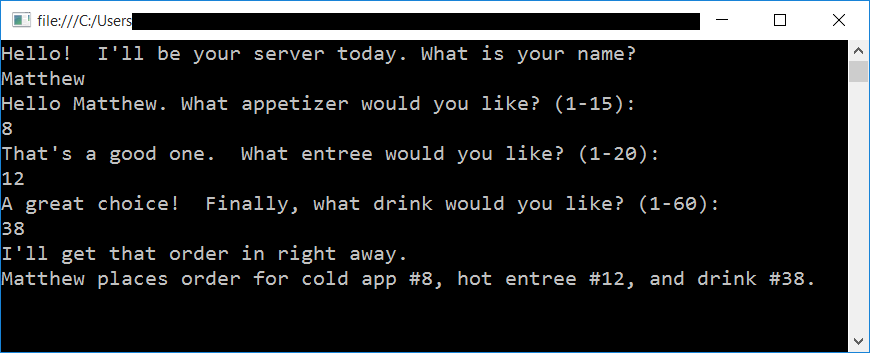
Will I Ever Need This Pattern?
All the time. Seriously, the Facade pattern is so general that it applies to almost every major app I've worked on, especially those where I couldn't refactor or modify pieces of said apps for various reasons.
Summary
The Facade pattern is a simple (or at least simpler) overlay on top of a group of more complex subsystems. The Facade knows which Subsystem to direct different kinds of work toward. And it's also really, really common, so it's one of the patterns we should know thoroughly.
Happy Coding!