My team recently had a new requirement in one of our projects: We needed to display a message to the users after they did some kind of action, but that message needed to disappear before the next request. We tried to do this using Session, but that didn't work out very well; after all, there's another construct we can use that does exactly this kind of thing: TempData
.
TempData
stores data placed into it until either you read it or until the completion of the next request, whichever is first.
But, of course, I wanted our solution to be a little more flexible that just having a bunch of TempData[]
checks all over my views and controllers. So, I now present to you: FlashMessage.
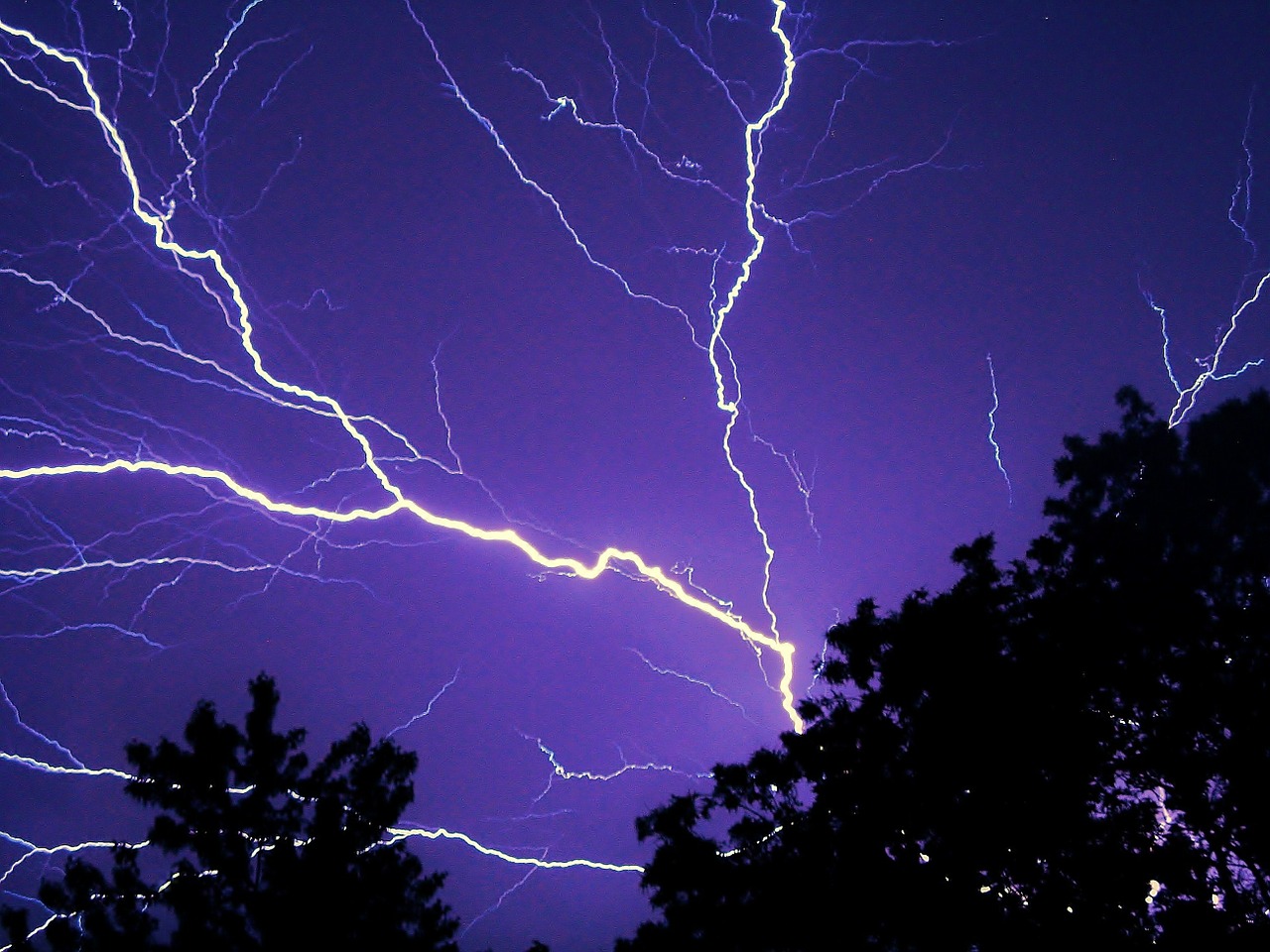
The Parts
First, there's an Enum for the types of message we can display:
public Enum FlashMessageType
{
Success,
Warning,
Error
}
We wanted this to be set on the controllers, but to do that they would need some way to share that property. So, let's make a ControllerBase
class:
public class ControllerBase : Controller
{
public void SetFlash(FlashMessageType type, string text)
{
TempData["FlashMessage.Type"] = type;
TempData["FlashMessage.Text"] = text;
}
}
We will have all the other controllers that need the FlashMessage
implementation inherit from ControllerBase
.
We still need to display the message. So, let's make a new partial view, FlashMessage.cshtml:
@{
string text = (string)TempData["FlashMessage.Text"];
string cssClass = null;
if (!string.IsNullOrWhiteSpace(text))
{
FlashMessageType type = (FlashMessageType)TempData["FlashMessage.Type"];
cssClass = type.ToString().ToLower();
}
}
@if (!string.IsNullOrWhiteSpace(text))
{
<div class="@cssClass">
<p>
@text
</p>
</div>
}
Now we can just call this on our main view like this: @Html.Partial("FlashMessage")
.
Finally, we set the message on the controller:
public class HomeController : ControllerBase
{
[HttpGet]
public ActionResult Index()
{
HomeIndexVM model = new HomeIndexVM();
return View(model);
}
[HttpPost]
public ActionResult Index(HomeIndexVM model)
{
SetFlash(model.Type, model.Message);
return RedirectToAction("Index");
}
}
And now we have a scalable, extensible Flash Message for our use anywhere in this project!
You can check out the sample project over on GitHub, and feel free to extend this however you like.
Happy Coding!