Over my years of blogging, I sometimes publish groups of stories that I call Deep Dives. These series consist of multiple related posts, and cover large or complicated topics, from design patterns to sorting algorithms to learning C#.
On this page, you can find all of my mega-series posts, including links to each part, a summary, more downloads, details, etc.
The individual series that comprise the Deep Dives are:
- C# In Simple Terms
- The Daily Design Pattern
- Modeling Practice (Board Games in C#)
- The Sorting Algorithm Family Reunion
- Designing a Workflow Engine Database
- Drawing with FabricJS and TypeScript
- Diary of a Death March
C# In Simple Terms
This series aims to introduce programmers to the C# language, including concepts, syntax, features, and more. It is intentionally written in the simplest possible language (which makes it perfect for newcomers to C# or people who do not speak English as a first language), and each post includes a glossary that defines terms used in that post.
The introductory post for this series is here:
eBook Available
You can also get C# In Simple Terms as an eBook by becoming a paid subscriber. The eBook even includes a bonus chapter about asynchronous programming that was not published on the site. Become a subscriber today!
The Daily Design Pattern
In this Deep Dive series, we explore how to implement Software Design Patterns using C#.
The introductory post for this series is here:
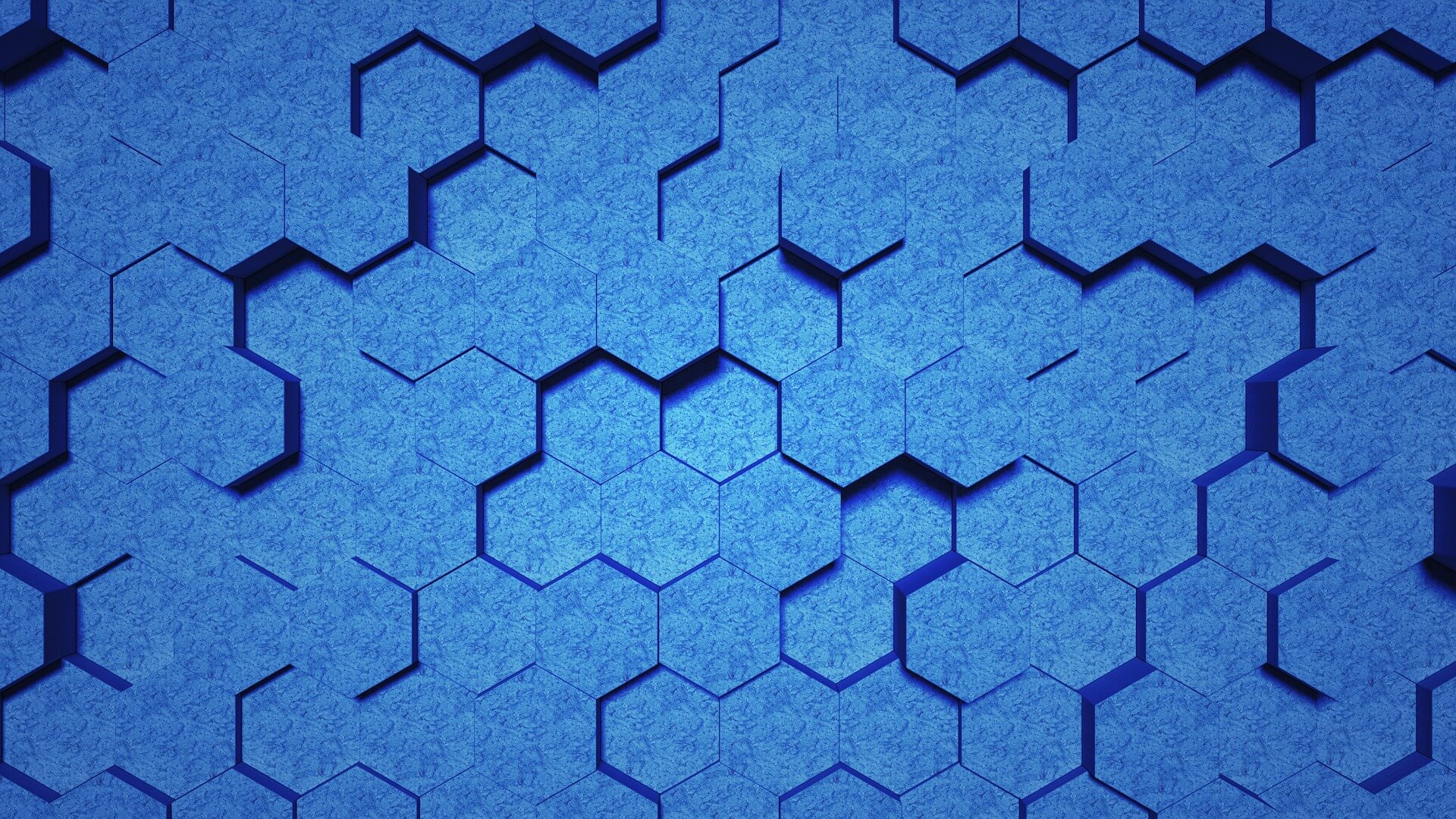
This series covers the following design patterns:
- Abstract Factory
- Adapter
- Bridge
- Builder
- Chain of Responsibility
- Command
- Composite
- Decorator
- Facade
- Factory Method
- Flyweight
- Iterator
- Mediator
- Memento
- Observer
- Prototype
- Proxy
- Singleton
- State
- Strategy
- Template Method
- Visitor
eBook Available
You can also get The Daily Design Pattern as an eBook by becoming a paid subscriber.
Modeling Practice
This Deep Dive series aims to give readers a change to practice their real-world modeling skills by taking known problems and designing them as C# applications.
One kind of real-world problem that must have a clearly-defined set of rules is board games. So, this series shows how to model certain board, card, and video games as C# applications.
The games we have modeled so far in this series include:
Candy Land
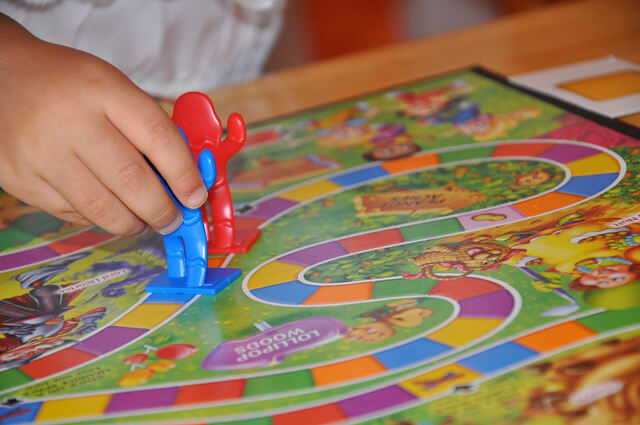
Uno
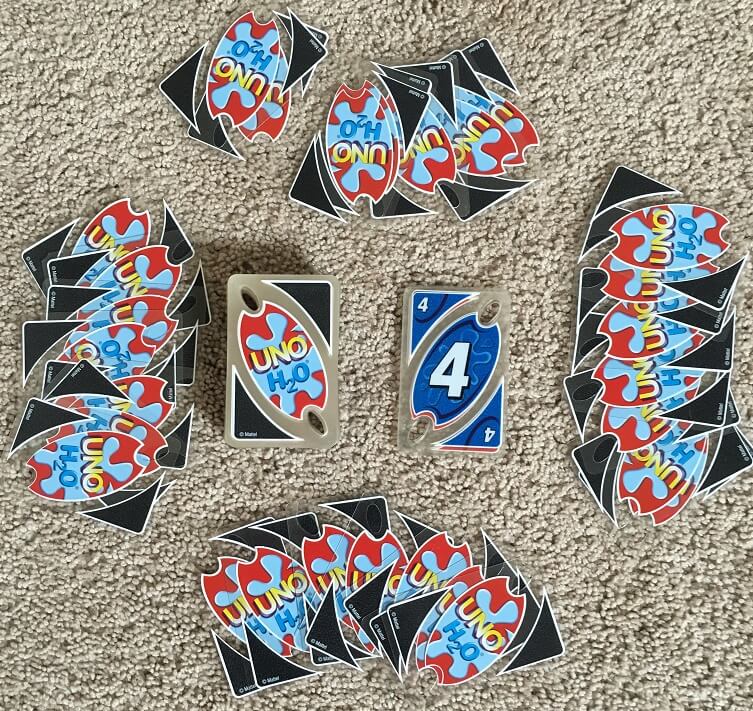
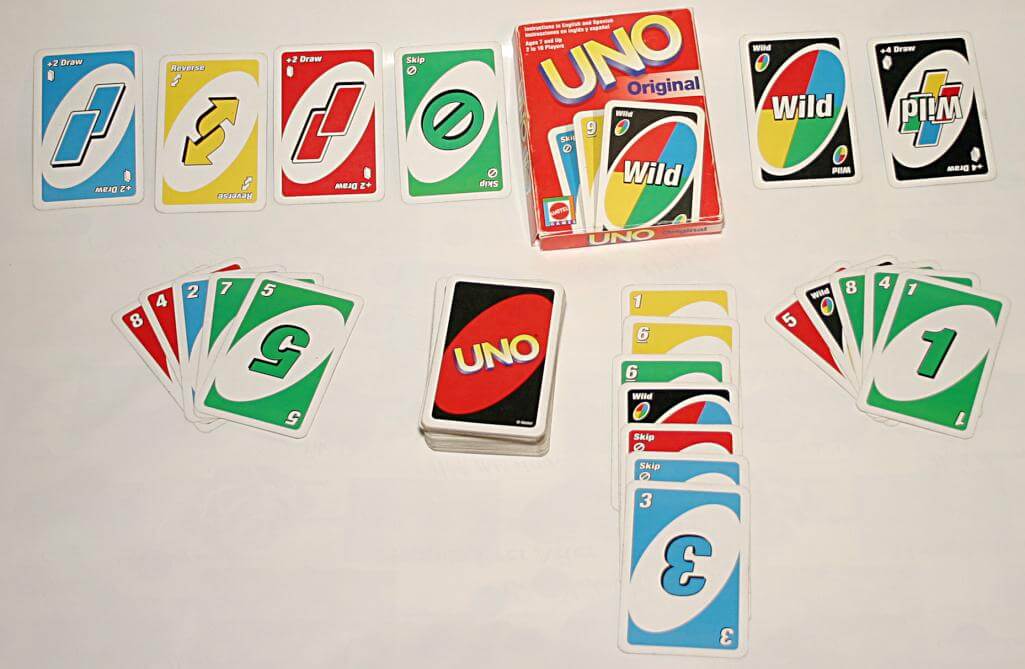
Minesweeper
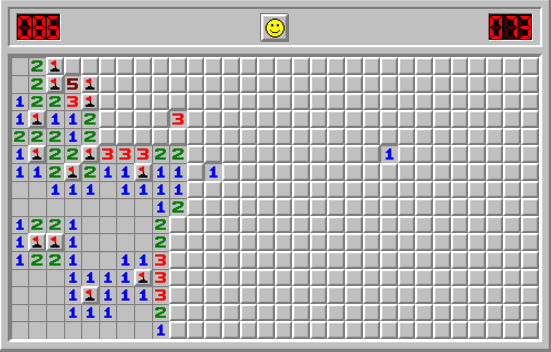
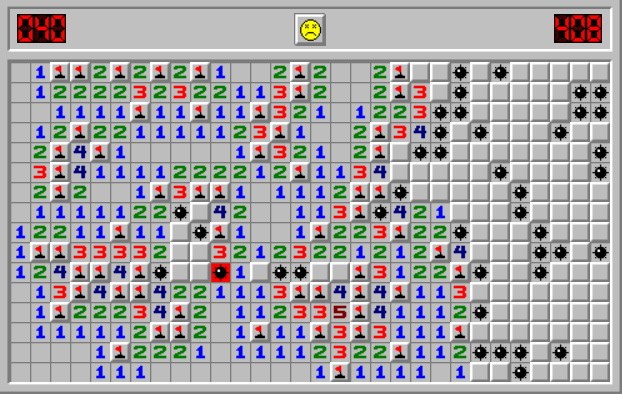
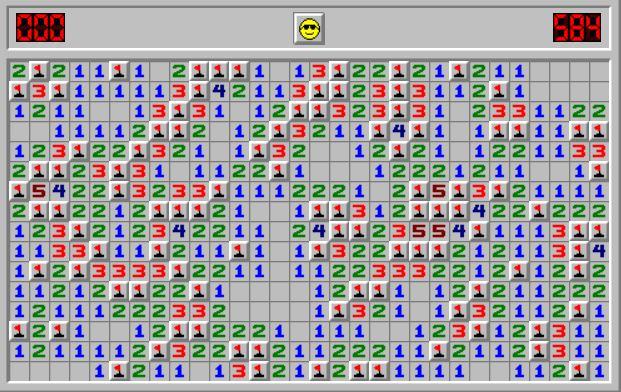
Battleship
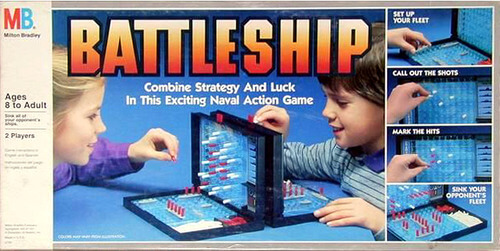
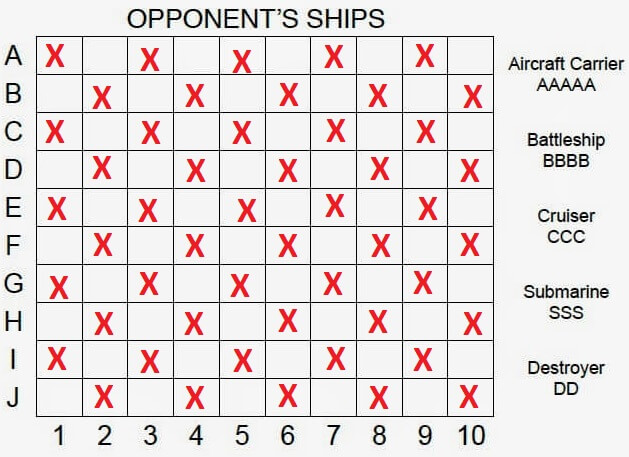
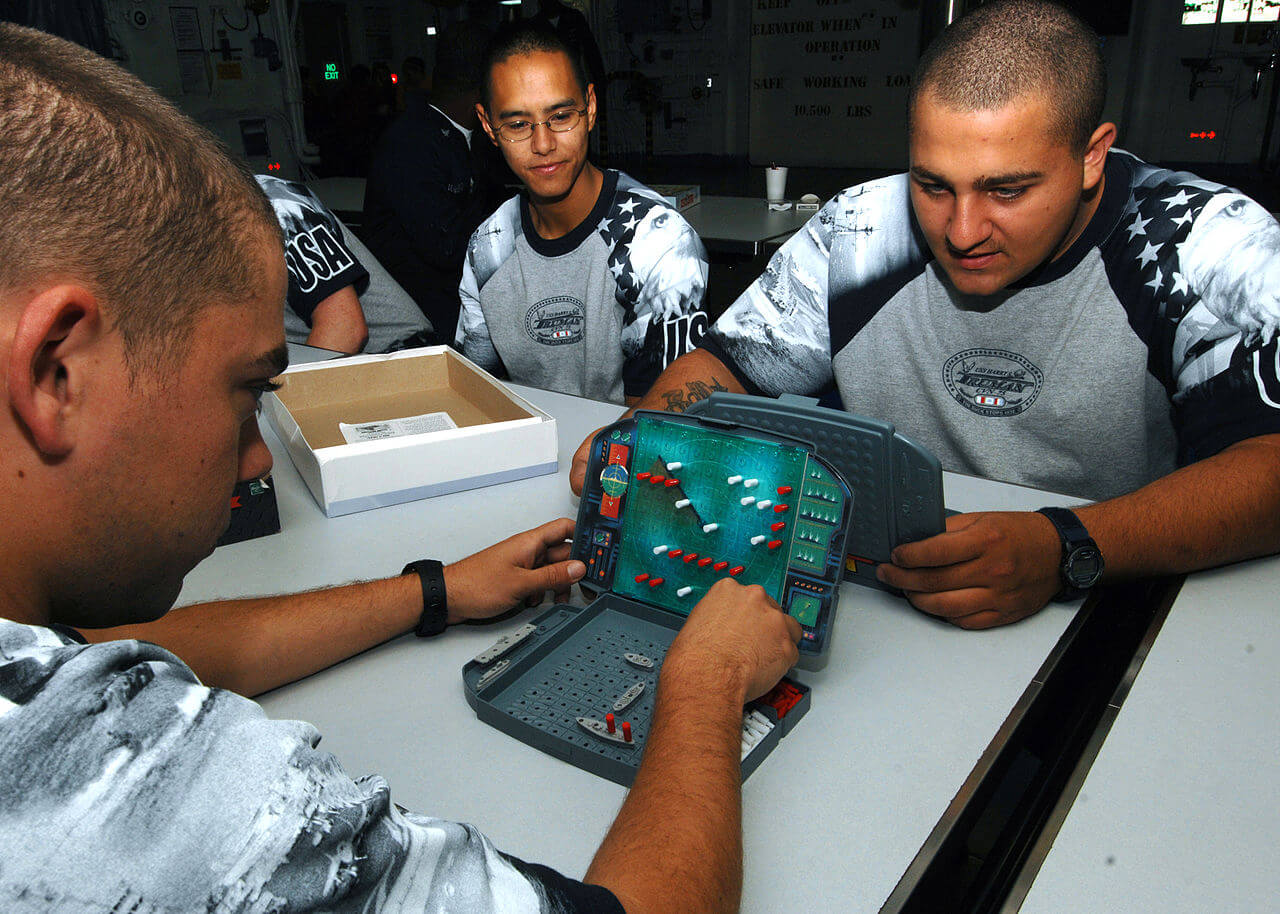
War (card game)
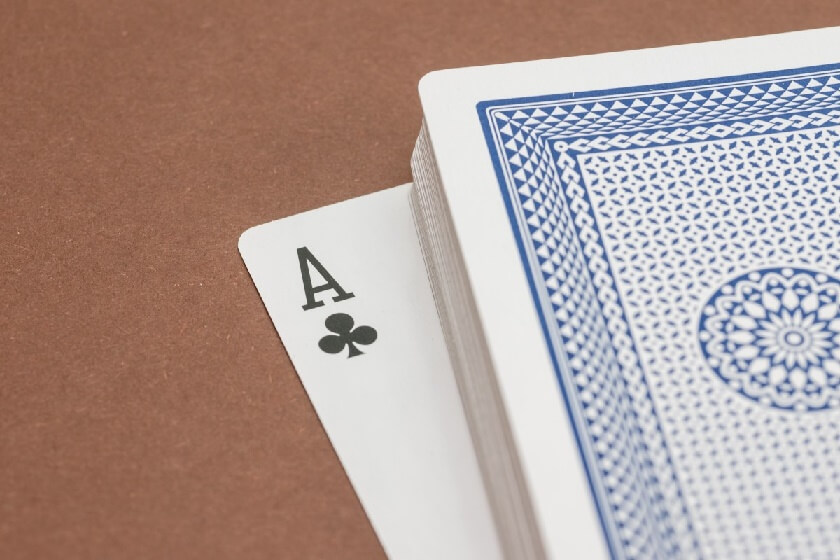
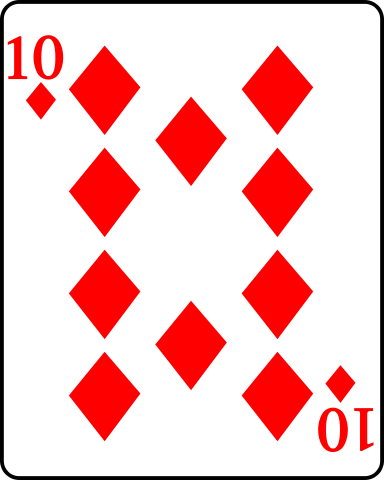
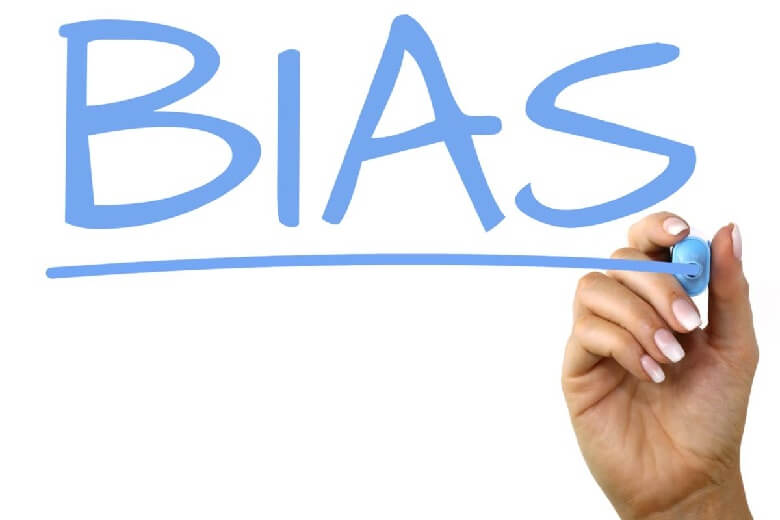
Tic-Tac-Toe
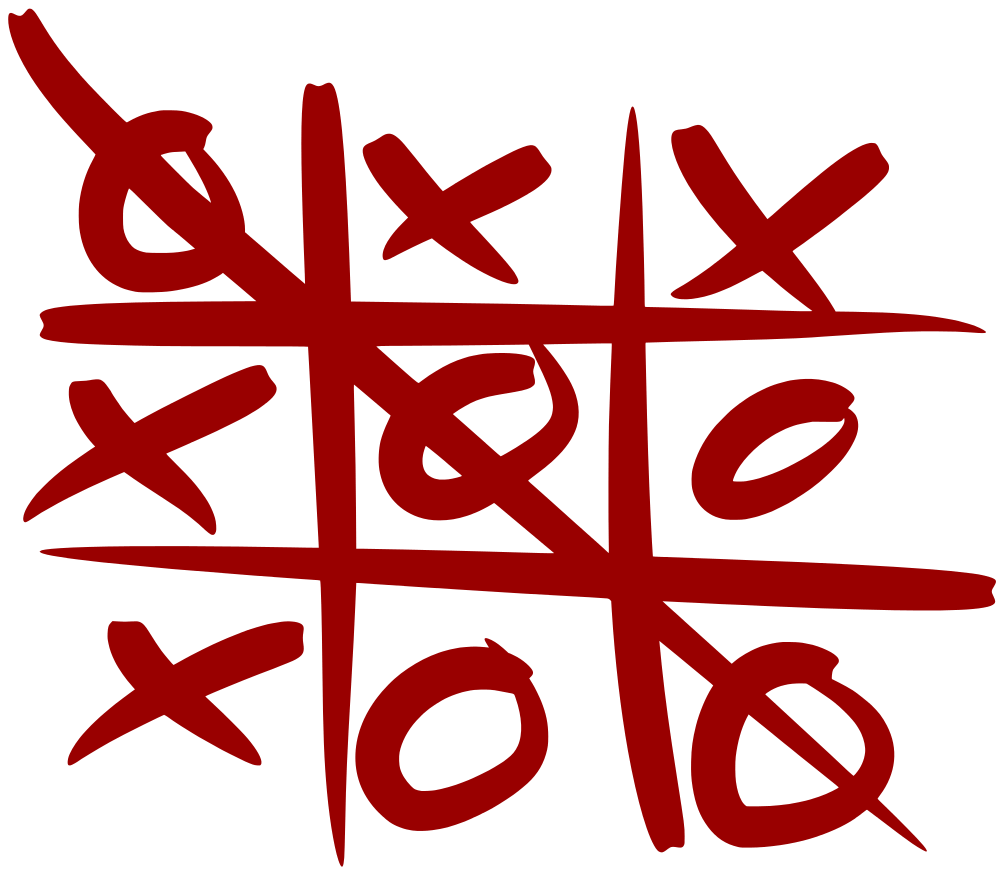
ConnectFour
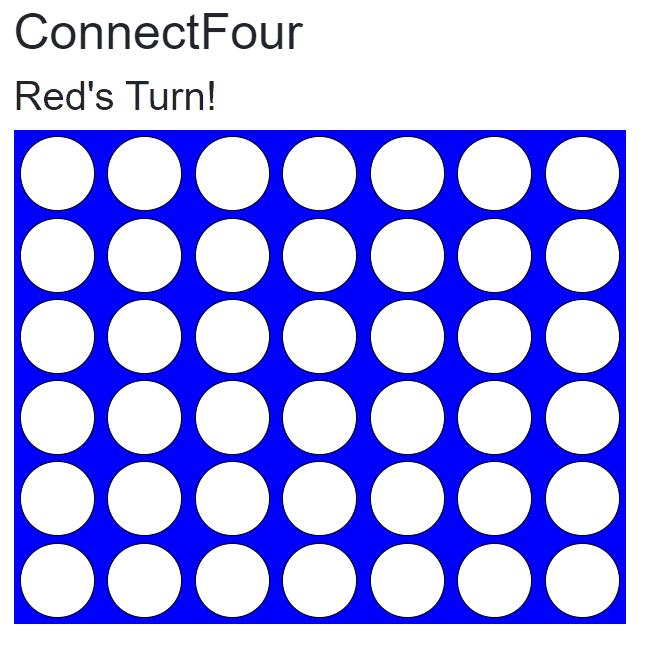
Ticket to Ride
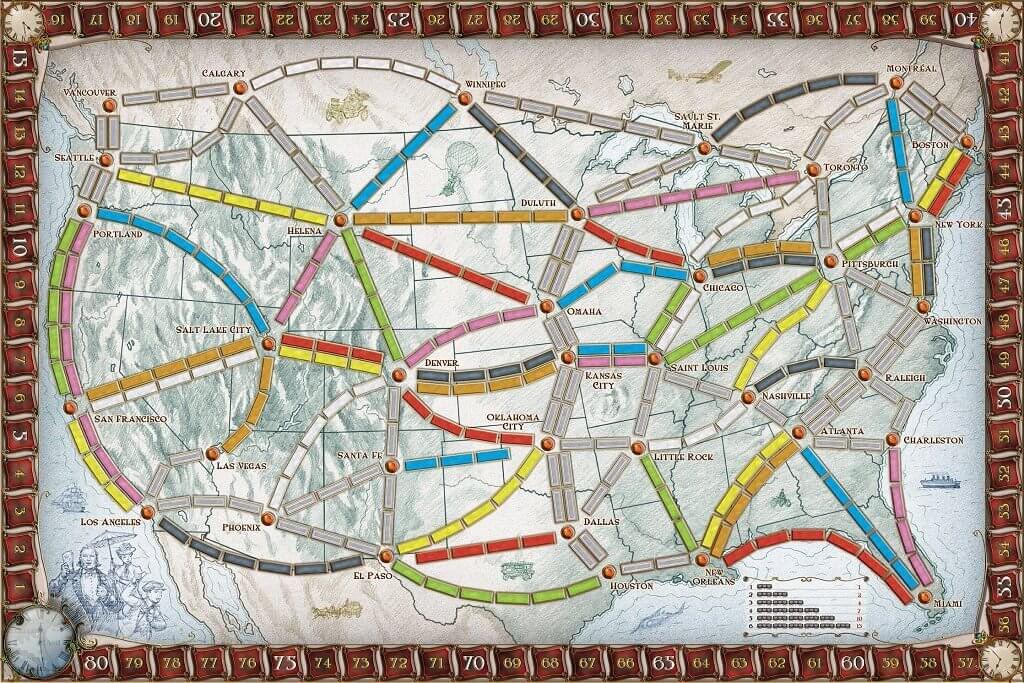
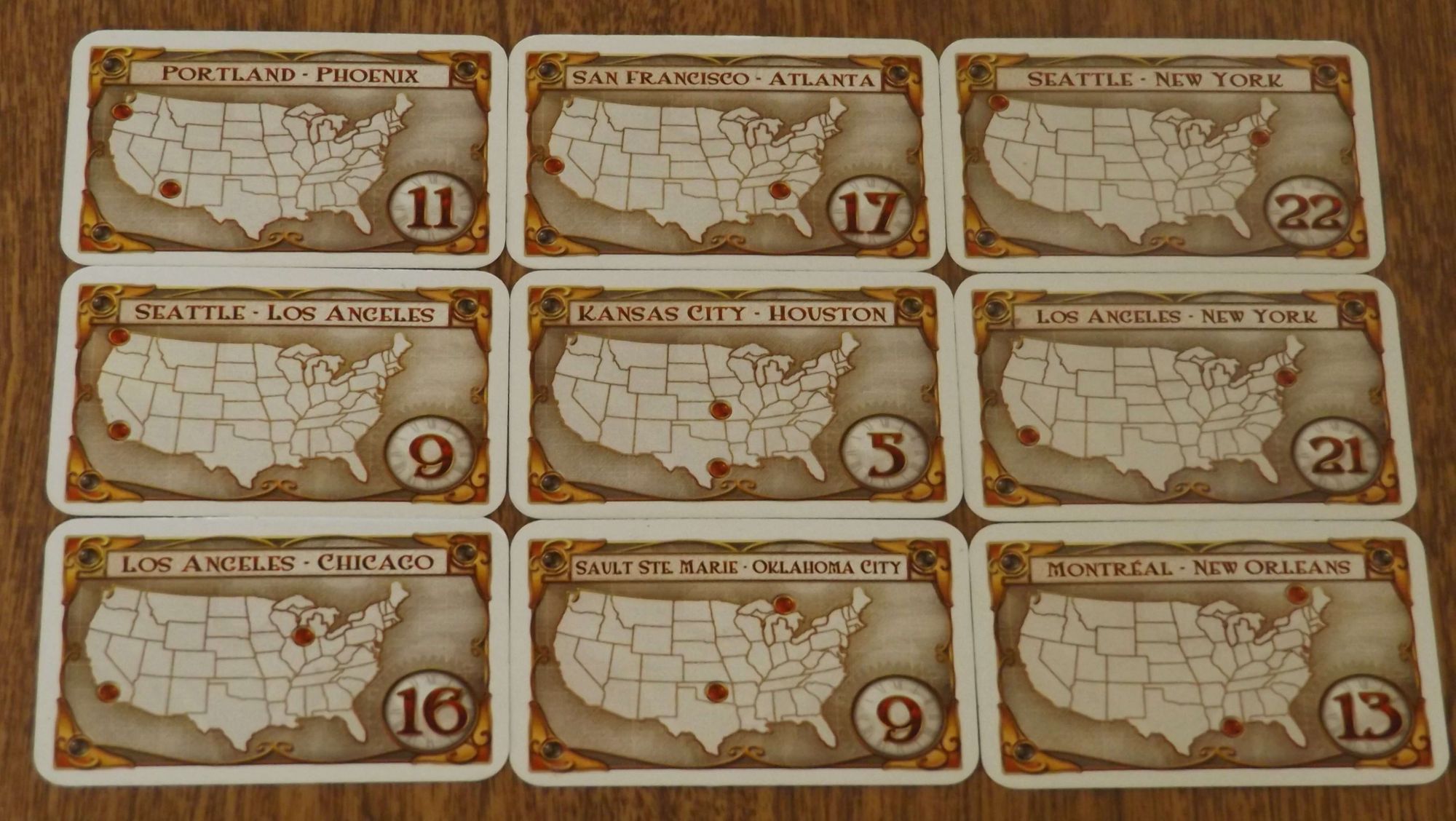
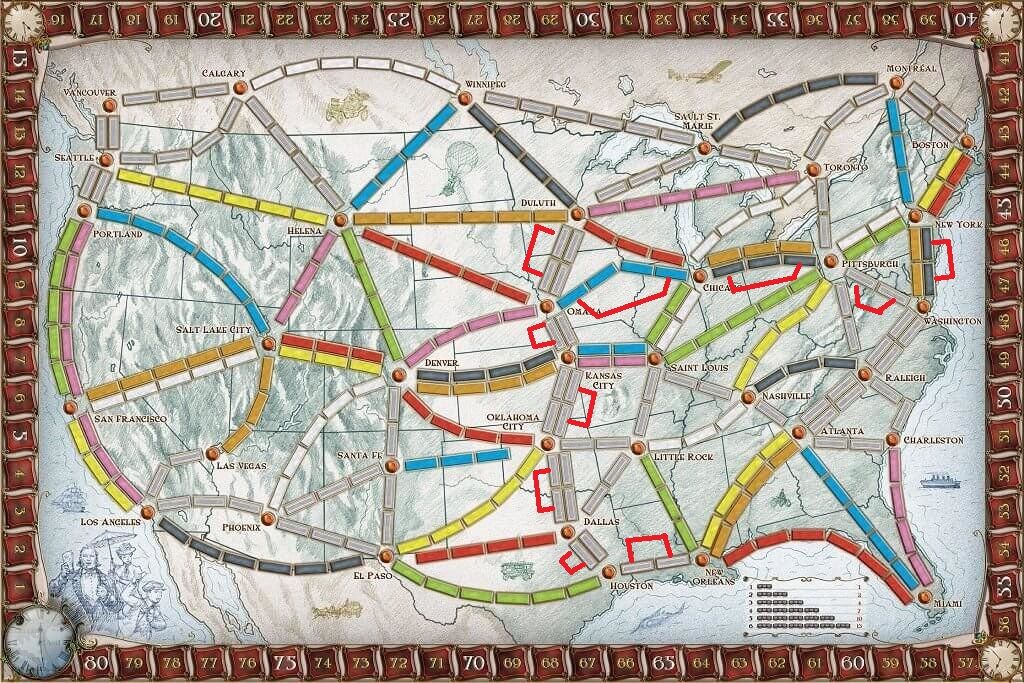
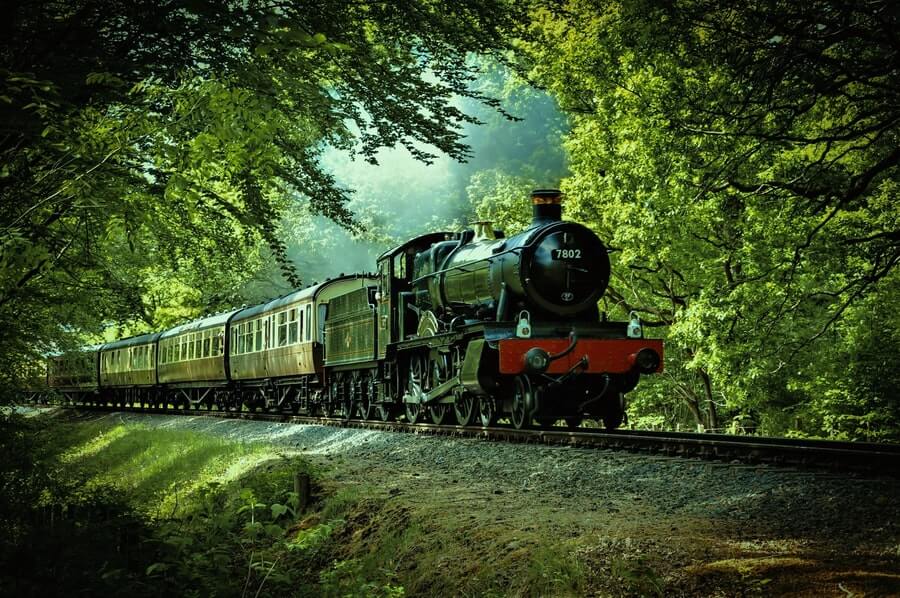
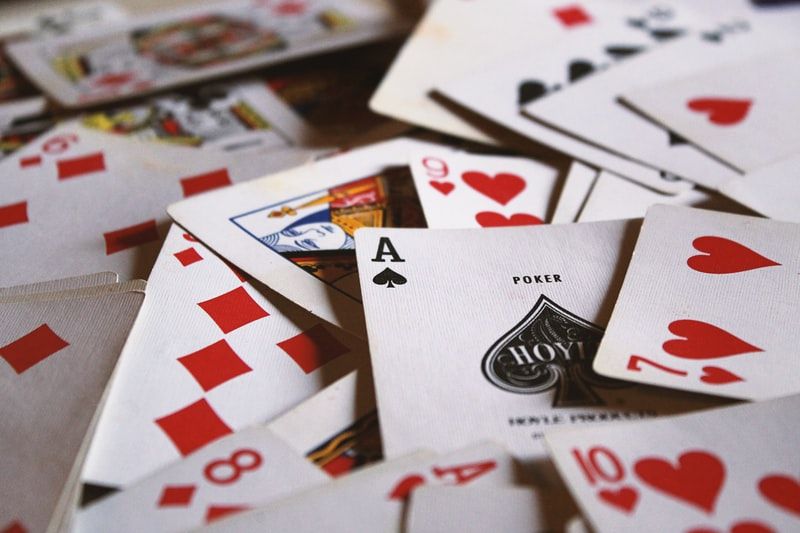
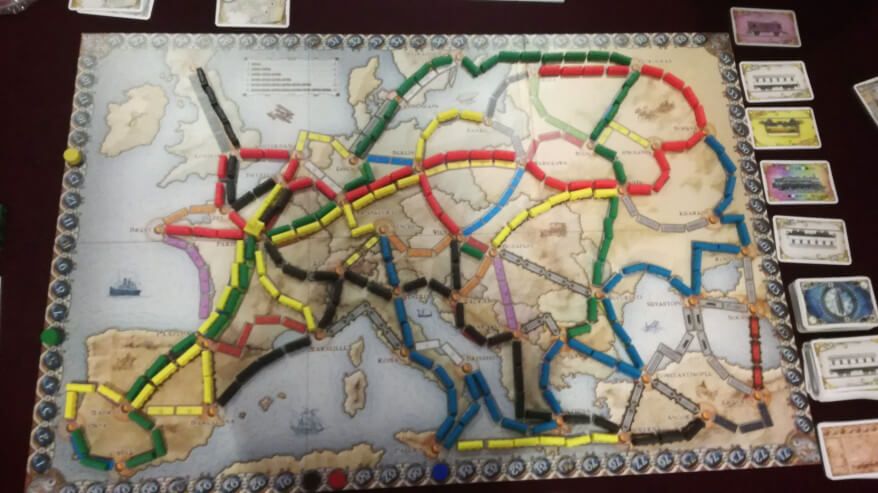
Conway's Game of Life
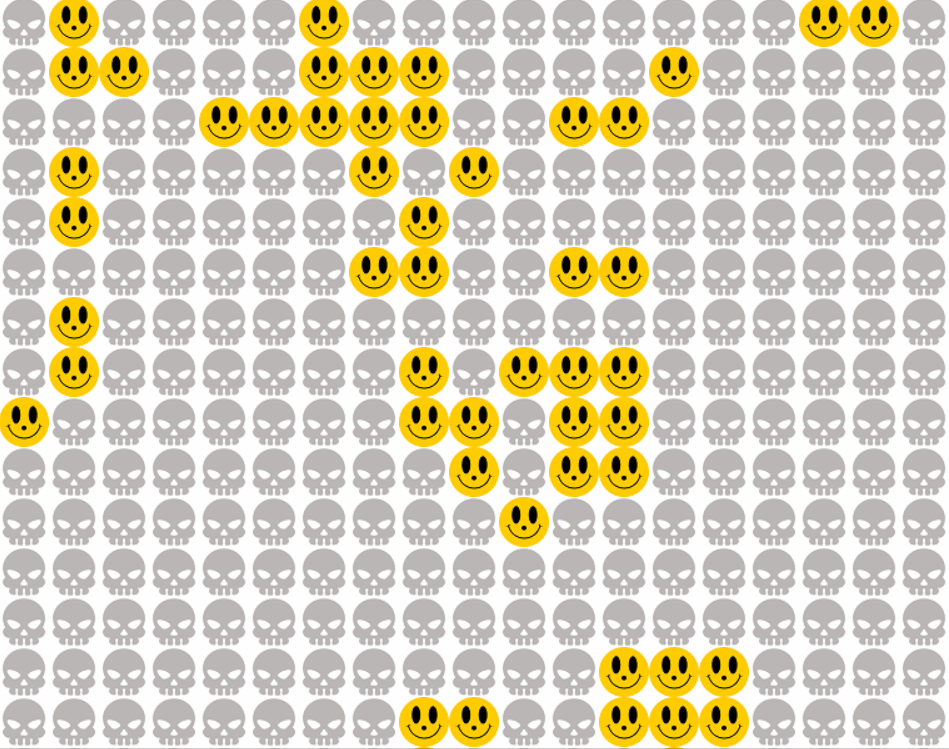
Yahtzee
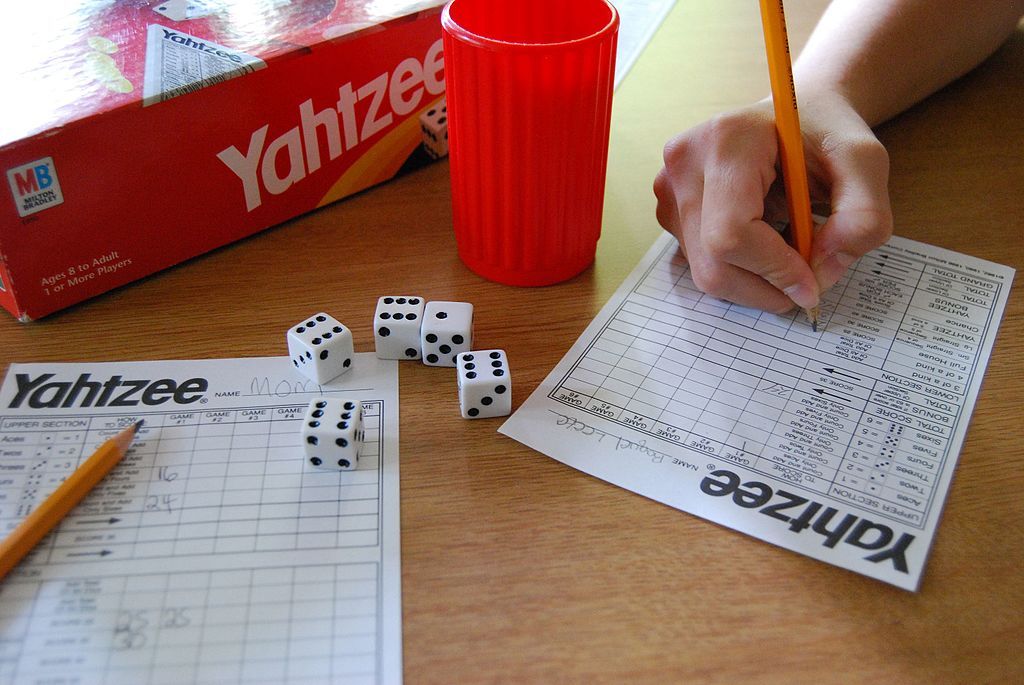
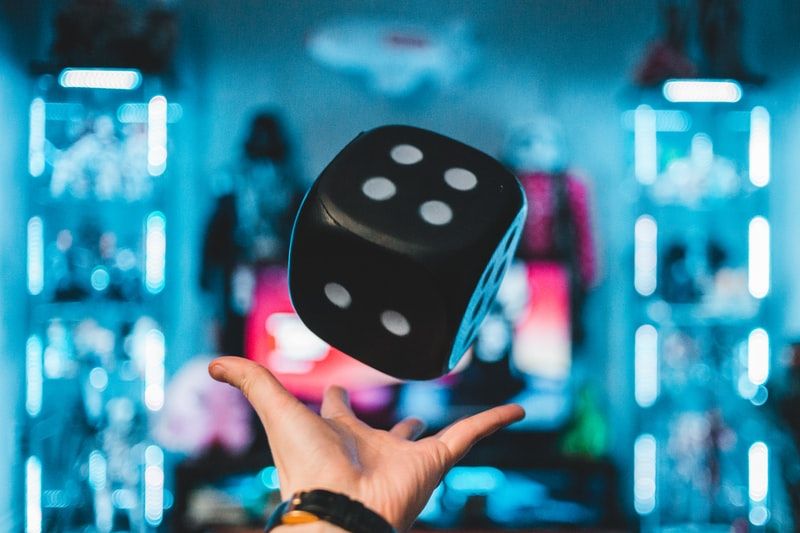
The Daily Software Anti-Pattern
As a counterpart to the Daily Design Pattern series, this series examine software anti-patterns and how to mitigate them.
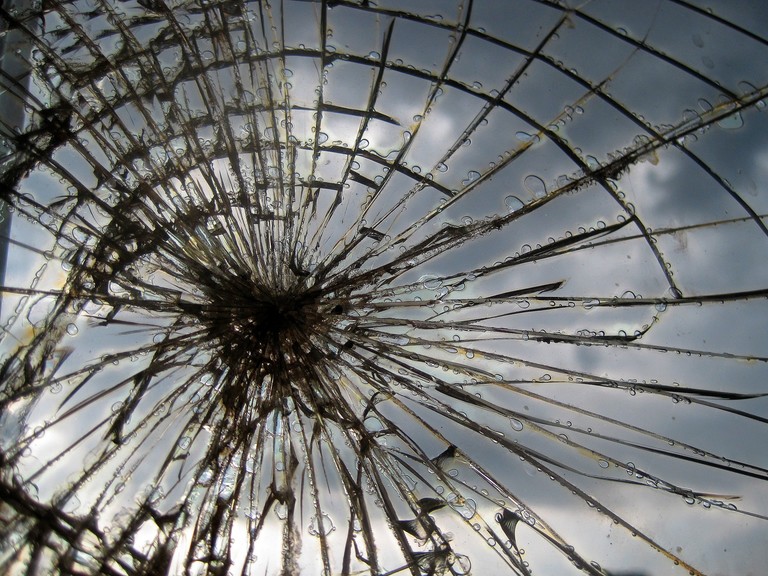
The introductory post for this Deep Dive is here:
The anti-patterns we discuss in this series include:
- The Inner-Platform Effect
- Spaghetti Code
- Not Invented Here
- Analysis Paralysis
- Reinventing the Square Wheel
- Lava Flow
- Stovepipe Enterprise
- God Objects
- Death by Planning
- Cargo Cult Programming
- Big Ball of Mud
- Boat Anchor
- Golden Hammer
- Gold Plating
- Bikeshedding
The Sorting Algorithm Family Reunion
This Deep Dive aims to explain and show how to implement many common sorting algorithms by imagining them as members of an extended family at a family reunion. In the process, we examine which of the algorithms are efficient and which aren't, and explain why this is. This series is quite possibly the nerdiest thing I have ever blogged about.
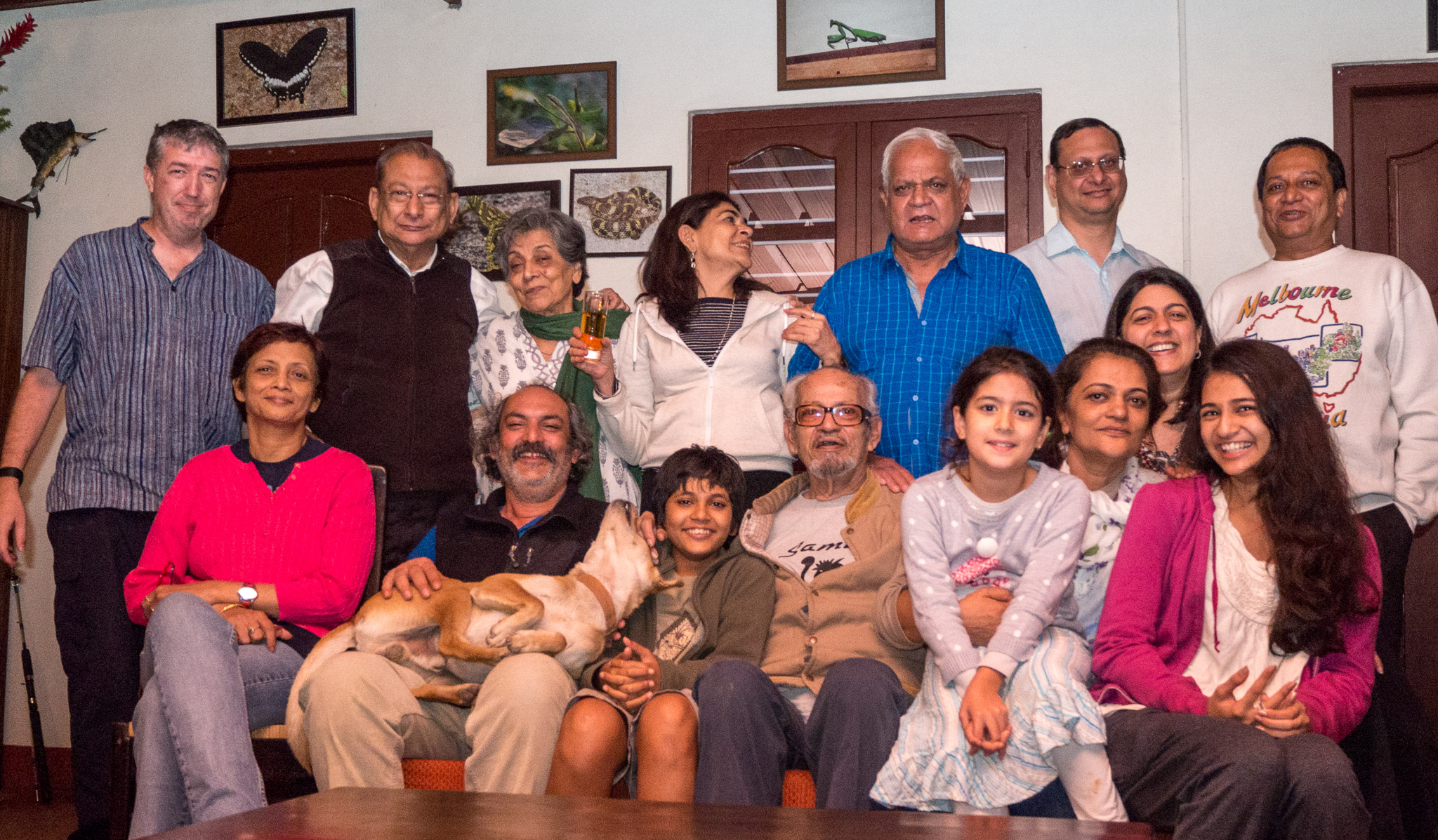
The introductory post for this Deep Dive series is here:
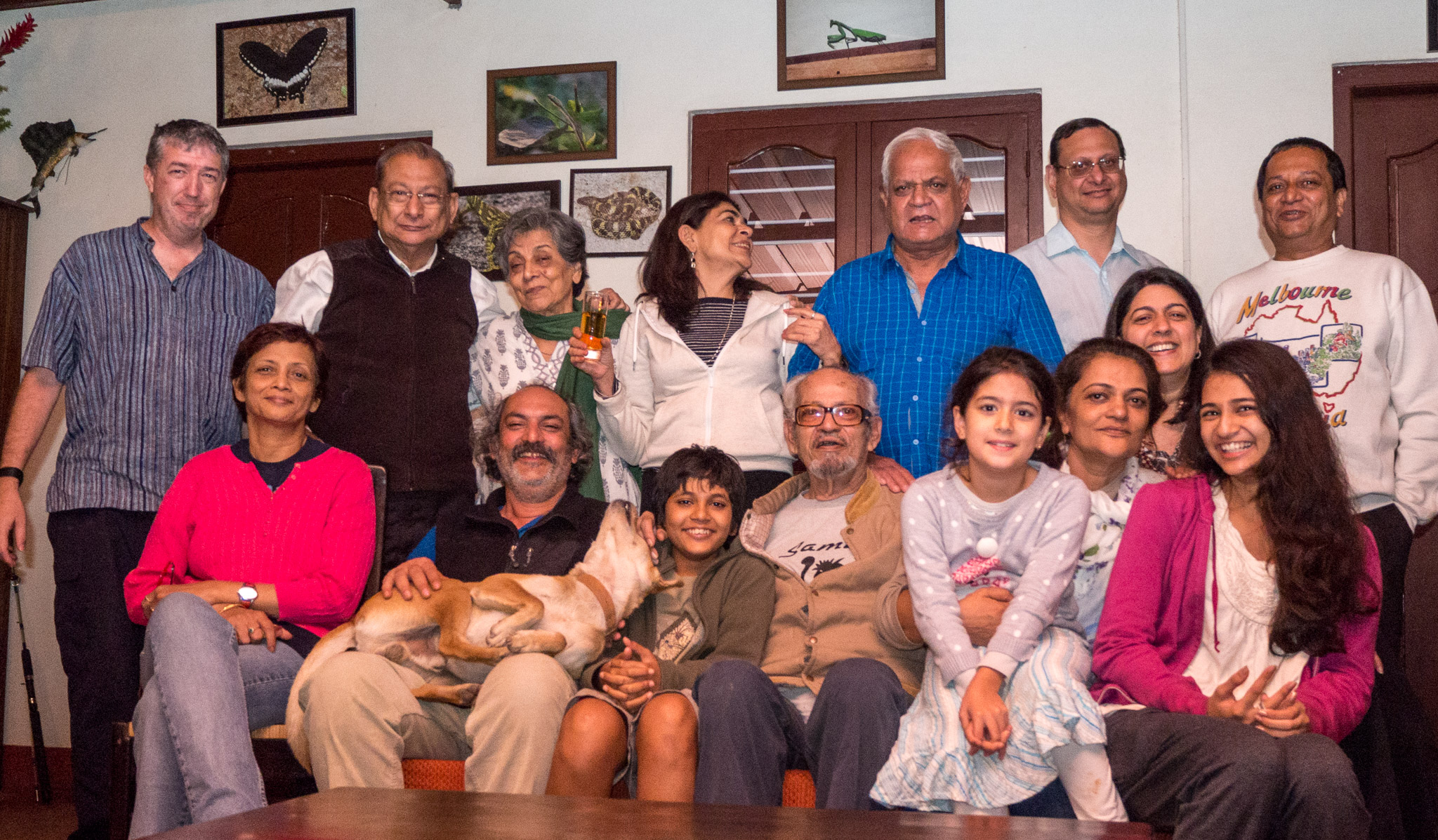
This series covers the following sorting algorithms:
- Selection Sort
- Merge Sort
- Insertion Sort
- Shell Sort
- Comb Sort
- Quick Sort
- Heap Sort
- Cocktail Shaker Sort
- Bogosort
- Bubble Sort
- Odd-Even Sort
- Counting Sort
- Bitonic Merge Sort
- Pigeonhole Sort
- Gnome Sort
- Radix Sort
- Bucket Sort
Designing a Workflow Engine Database
This series explores how to make a workflow-style database in SQL. We discuss what tables and objects we need, how they are related to each other, and how requests flow through the engine.
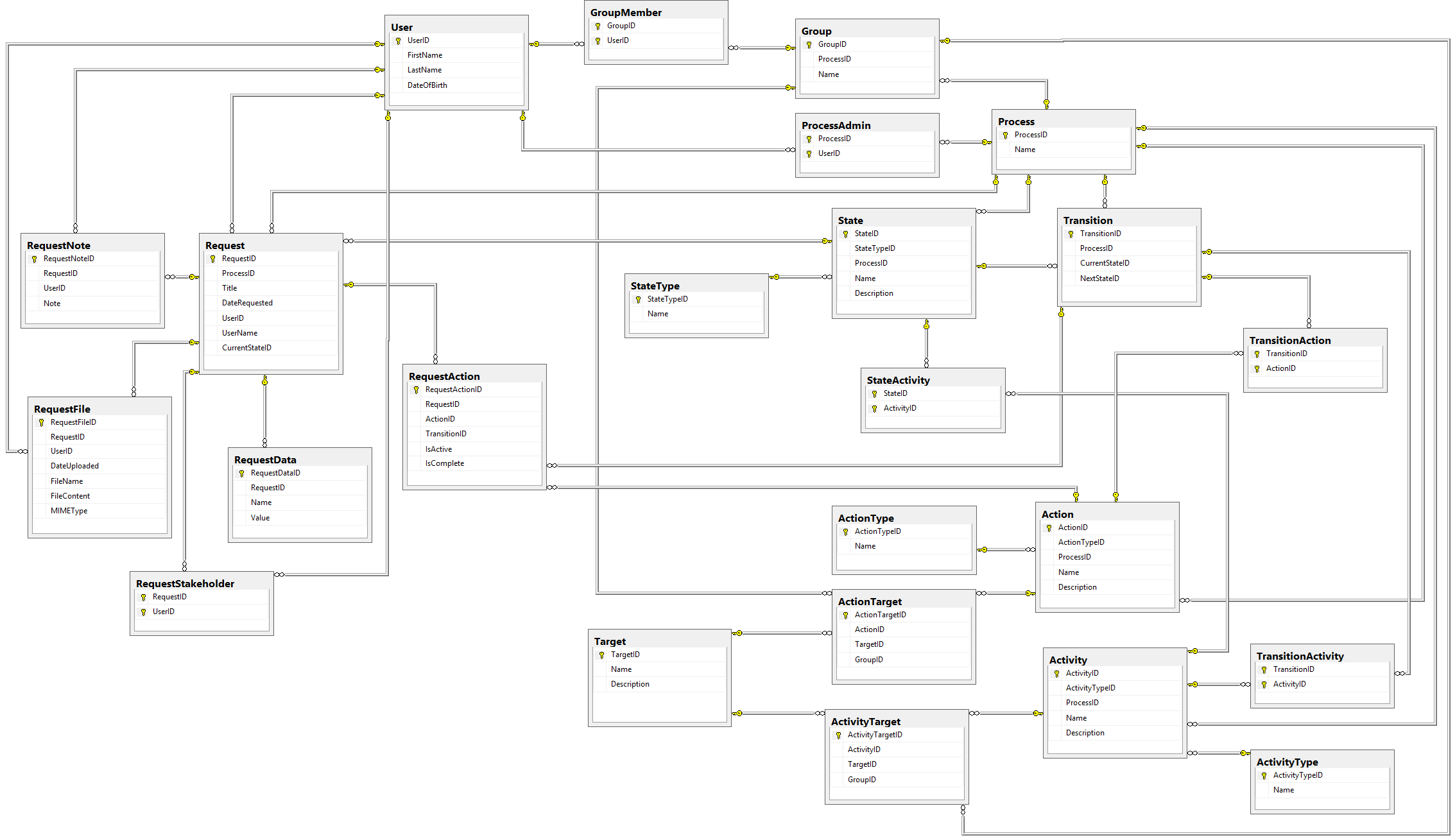
You can start this Deep Dive series at Part 1 here:
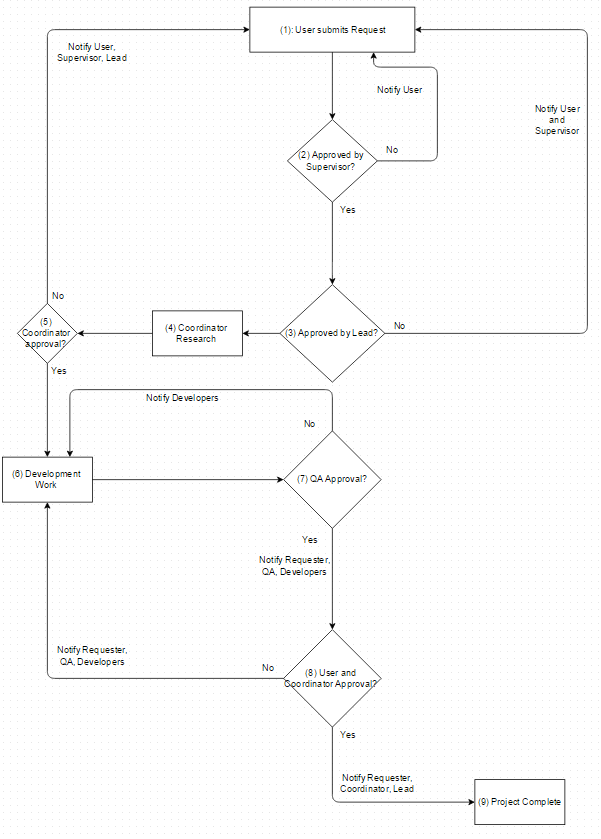
The rest of the series includes the following:
- Part 2: The Process Table and Users
- Part 3: Request Details and Data
- Part 4: States and Transitions
- Part 5: Actions and Activities
- Part 6: Groups and Targets
- Part 7: Request Actions
- Part 8: Complete Schema and Shortcomings
Drawing with FabricJS and TypeScript
This series explores how to use the FabricJS library to make a drawing application in TypeScript.
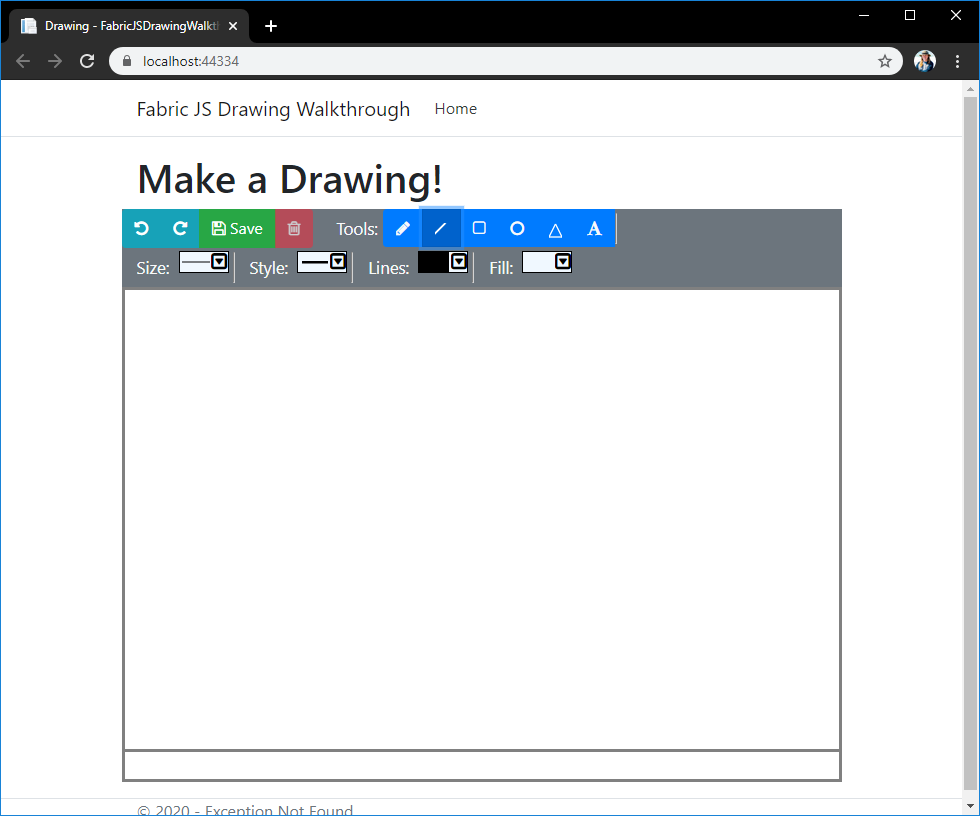
You can start this series with Part 1: Intro, Goals, and Setup here:
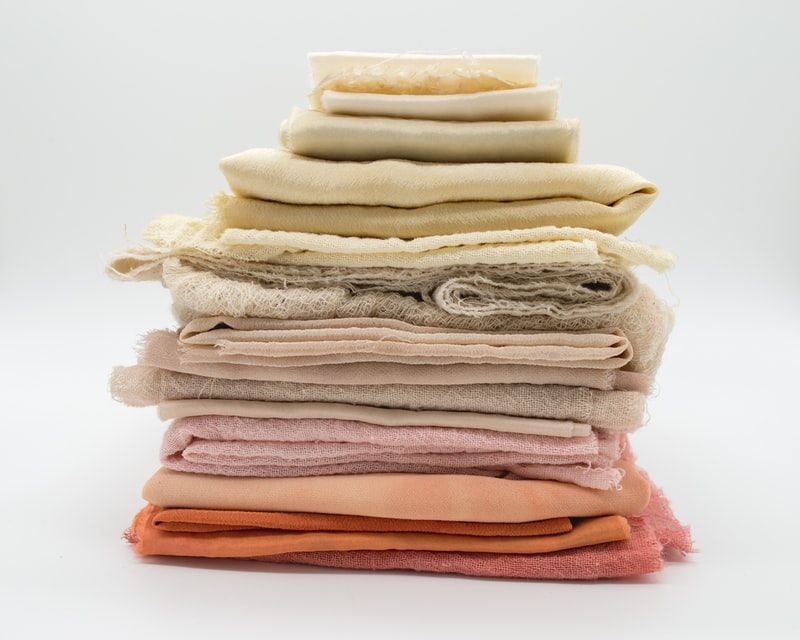
The rest of the series includes the following posts:
- Part 2: Straight Lines
- Part 3: Basic Shapes
- Part 4: Text and Freeform Lines
- Part 5: Deleting Objects
- Part 6: Colors and Styles
- Part 7: Undo/Redo
- Part 8: Cut/Copy/Paste and Hotkeys
- Part 9: Saving and Conclusion
Diary of a Death March
Diary of a Death March is a series of short stories I wrote about a company that was forcing its employees onto a "death march". The stories are written from different points of view, and with different characterizations. This series is a work of fiction.
The posts in this series are: